[Link]
Password:
tool.py
AoB Extractor:
Spoiler
Code: Select all
# Original code with predefined byte sequences
original_code_with_brackets = """
"""
def extract_aob_from_original_code(original_code):
aob = "" # AOB after the injection section
before_injecting = "" # Bytes before the injection section
start_injecting = False # Flag for when we are in the injection section
in_original_code = False # Flag to mark entry into the original section
# Iterate over the lines of the original code
for line in original_code.split('\n'):
line = line.strip() # Remove leading and trailing spaces
# Ignore empty lines or non-relevant general comments
if not line or line.startswith('{') or line.startswith('}') or 'Game' in line or 'Version' in line or 'Date' in line or 'Author' in line or 'This script does' in line:
continue
# Start of the injection section
if "// ---------- INJECTING HERE ----------" in line:
start_injecting = True
continue
# End of the injection section
if "// ---------- DONE INJECTING ----------" in line:
start_injecting = False
continue
# Ignore braces
if line == "{" or line == "}":
continue
# Check if the line contains an instruction with address recognition
if ':' in line and not line.startswith('//'):
parts = line.split(':')
# Ensure we have an address and consider only the first format
if len(parts) > 1 and parts[0].strip():
byte_str = parts[1].strip().split('-')[0].strip() # Get the byte part
if start_injecting:
# Add to the complete AOB
aob += byte_str + " "
else:
# Add to the bytes before the injection section
before_injecting += byte_str + " "
# Remove any extra spaces
aob = aob.strip()
before_injecting = before_injecting.strip()
# Calculate the number of bytes
before_injecting_bytes = len(before_injecting.split()) if before_injecting else 0 # Prevent errors
return aob, before_injecting, before_injecting_bytes
# Extract AOB from the codes
original_aob, before_injecting, before_injecting_bytes = extract_aob_from_original_code(original_code_with_brackets)
# Print the result
print("Before Injecting point Bytes:")
print(before_injecting)
print("Byte count:", before_injecting_bytes)
print(hex(before_injecting_bytes))
print(f"{before_injecting_bytes:X}")
print()
print("AOB from original code:")
print(original_aob)
print("Byte count:", len(original_aob.split())) # Calculate AOB bytes
print(hex(len(original_aob.split()))) # Hex value
# Calculate the AOB byte count
aob_bytes_count = len(original_aob.split())
print(f"{aob_bytes_count:X}")
print()
Video:
Spoiler
AoB Comparator:
Spoiler
Code: Select all
# Original code with predefined byte sequences
original_code_with_brackets_1 = """
"""
# Original code 2 with predefined byte sequences for comparison
original_code_with_brackets_2 = """
"""
def extract_aob_from_original_code(original_code):
aob = "" # AOB after the injection section
before_injecting = "" # Bytes before the injection section
start_injecting = False # Flag for when we are in the injection section
# Iterate over the lines of the original code
for line in original_code.split('\n'):
line = line.strip() # Remove leading and trailing spaces
# Ignore empty lines or non-relevant general comments
if not line or line.startswith('{') or line.startswith('}') or 'Game' in line or 'Version' in line or 'Date' in line or 'Author' in line or 'This script does' in line:
continue
# Start of the injection section
if "// ---------- INJECTING HERE ----------" in line:
start_injecting = True
continue
# End of the injection section
if "// ---------- DONE INJECTING ----------" in line:
start_injecting = False
continue
# Ignore braces
if line == "{" or line == "}":
continue
# Ensure we have an address instruction
if ':' in line and not line.startswith('//'):
parts = line.split(':')
# Ensure we have an address and consider only the first format
if len(parts) > 1 and parts[0].strip():
byte_str = parts[1].strip().split('-')[0].strip() # Get the byte part
if start_injecting:
# Add to the complete AOB
aob += byte_str + " "
else:
# Add to the bytes before the injection section
before_injecting += byte_str + " "
# Remove any extra spaces
aob = aob.strip()
before_injecting = before_injecting.strip()
# Calculate the number of bytes
before_injecting_bytes = len(before_injecting.split()) if before_injecting else 0 # Prevent errors
return aob, before_injecting, before_injecting_bytes
def compare_bytes(before_injecting1, before_injecting2):
bytes1 = before_injecting1.split()
bytes2 = before_injecting2.split()
max_len = max(len(bytes1), len(bytes2))
result = []
for i in range(max_len):
byte1 = bytes1[i] if i < len(bytes1) else "??"
byte2 = bytes2[i] if i < len(bytes2) else "??"
if byte1 == byte2:
result.append(byte1)
else:
result.append("??")
return ' '.join(result), len(result), len(bytes1), len(bytes2)
def compare_aobs(aob1, aob2):
bytes1 = aob1.split()
bytes2 = aob2.split()
max_len = max(len(bytes1), len(bytes2))
result = []
for i in range(max_len):
byte1 = bytes1[i] if i < len(bytes1) else "??"
byte2 = bytes2[i] if i < len(bytes2) else "??"
if byte1 == byte2:
result.append(byte1)
else:
result.append("??")
return ' '.join(result), len(result), len(bytes1), len(bytes2)
# Extract AOB from the codes for comparison (1)
original_aob1, before_injecting1, before_injecting_bytes1 = extract_aob_from_original_code(original_code_with_brackets_1)
# Extract AOB from the codes for comparison (2)
original_aob2, before_injecting2, before_injecting_bytes2 = extract_aob_from_original_code(original_code_with_brackets_2)
# Comparison of results
print("\n--- Compare Result ---\n")
# Compare the bytes before injection
comparison_before_injecting, comp_before_count, len_before1, len_before2 = compare_bytes(before_injecting1, before_injecting2)
print("Before Injecting point Bytes _ Compare Result:")
print(comparison_before_injecting)
print("Byte count:", comp_before_count)
print(hex(comp_before_count))
print(f"{comp_before_count:X}")
# Comparison of the AOBs
comparison_aob, comp_aob_count, len_aob1, len_aob2 = compare_aobs(original_aob1, original_aob2)
print("\nAOB from original code _ Compare Result:")
print(comparison_aob)
print("Byte count:", comp_aob_count)
print(hex(comp_aob_count))
print(f"{comp_aob_count:X}")
# Warnings for different lengths
warning_before = False # Flag for warning the bytes before injection
if len_before1 != len_before2:
print("\n------ _WARNING_ ------")
print("Before Injecting point bytes have different lengths.")
print("------ _WARNING_ ------\n")
warning_before = True
# Warning for AOB length
warning_aob = False # Flag for warning the AOBs
if len_aob1 != len_aob2:
print("\n------ _WARNING_ ------")
print("AOB arrays have different lengths.")
print("------ _WARNING_ ------\n")
warning_aob = True
# If there are no warnings, do not print further
if not warning_before and not warning_aob:
print()
print("\nNo warnings. All byte comparisons match.\n")
Video:
Spoiler
IDE:
Spoiler
[Link]
I place it here because I don't know where to post it.
A simple script for Python 3 to extract AoB from a CE script.
Code: Select all
code = """
"""
aob = ''.join([line.split(':')[1].split('-')[0].strip().replace(' ', '') for line in code.split('\n') if line.strip() != '' and not line.strip().startswith('//')])
formatted_aob = ' '.join([aob[i:i + 2] for i in range(0, len(aob), 2)])
print(formatted_aob)
Premise this does not work if you have the opportunity to see the modules activated.
Guide to disable it:
First of all you have to click on "Memory View", then on "View" and then you have to remove the check from "Show Symbols" (I recommend reactivating the tick after use).
(There is no need to do this for all games depends if the game uses Modules or Mono, in that case you do.)
Spoiler
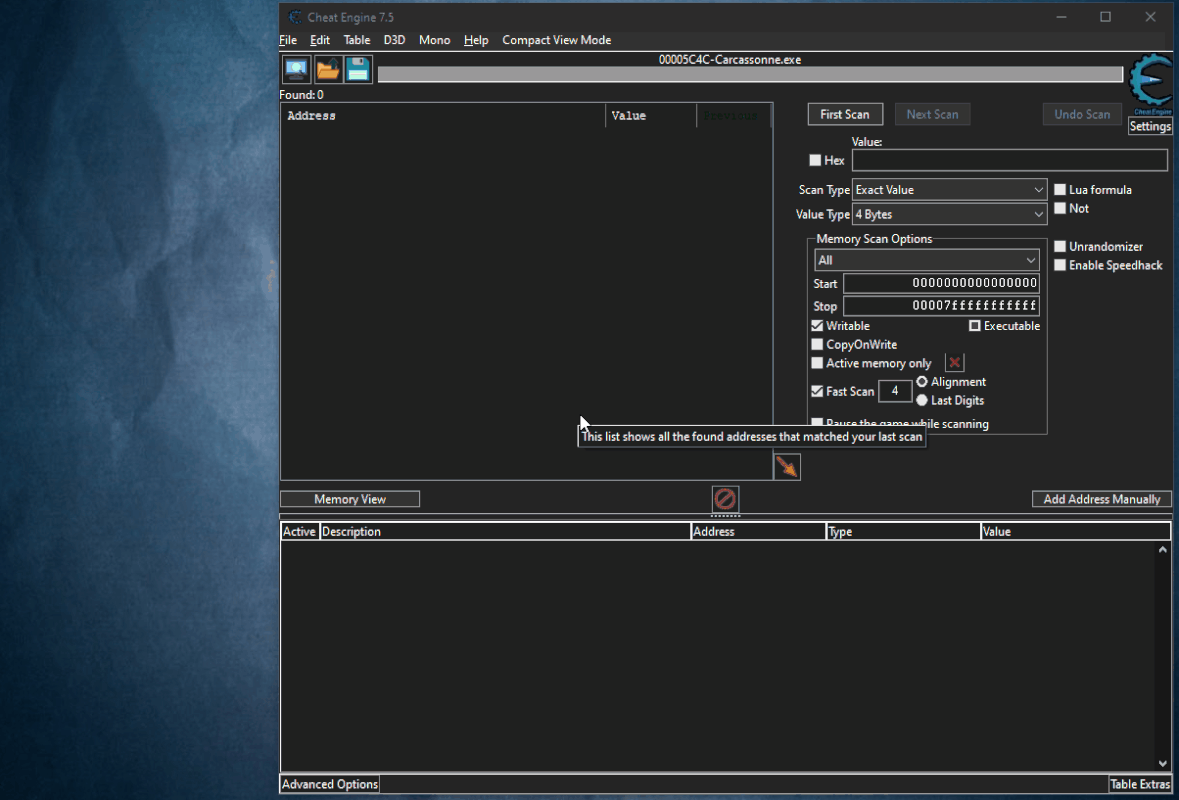
Here are the steps to be made:
Open the script go all the way and select the piece of code from which you want to extract the AoB. Copy the code and paste between
Code: Select all
code = """
"""
Spoiler

Spoiler
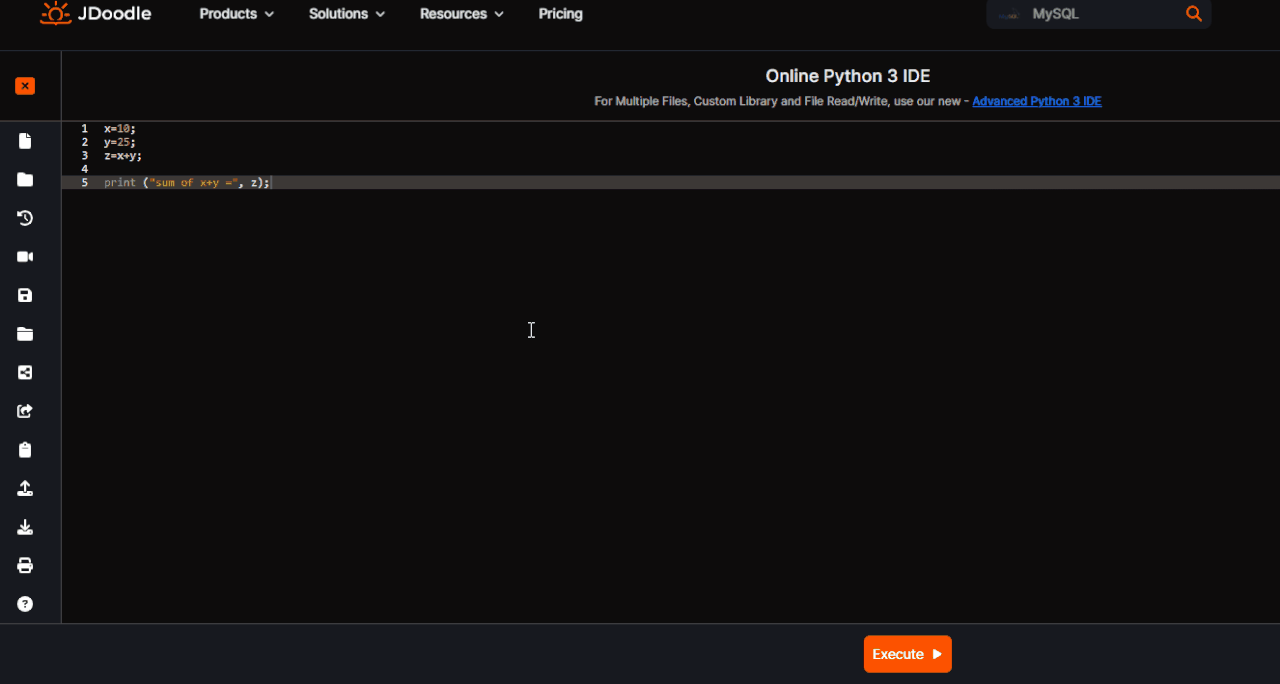
Spoiler
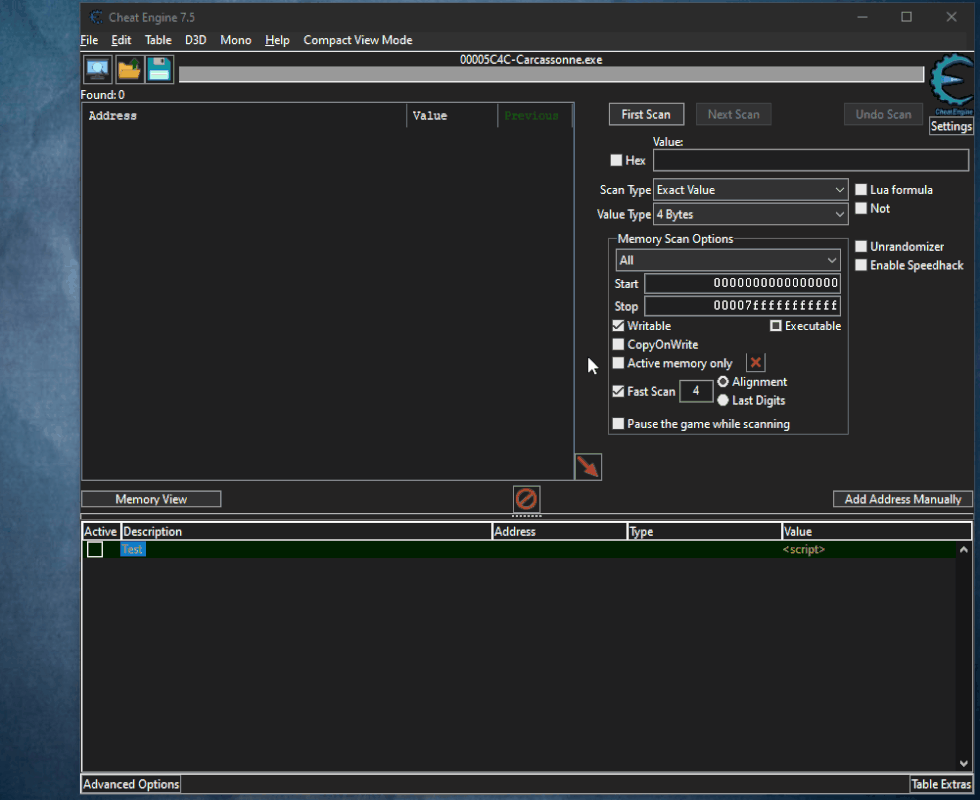
Spoiler
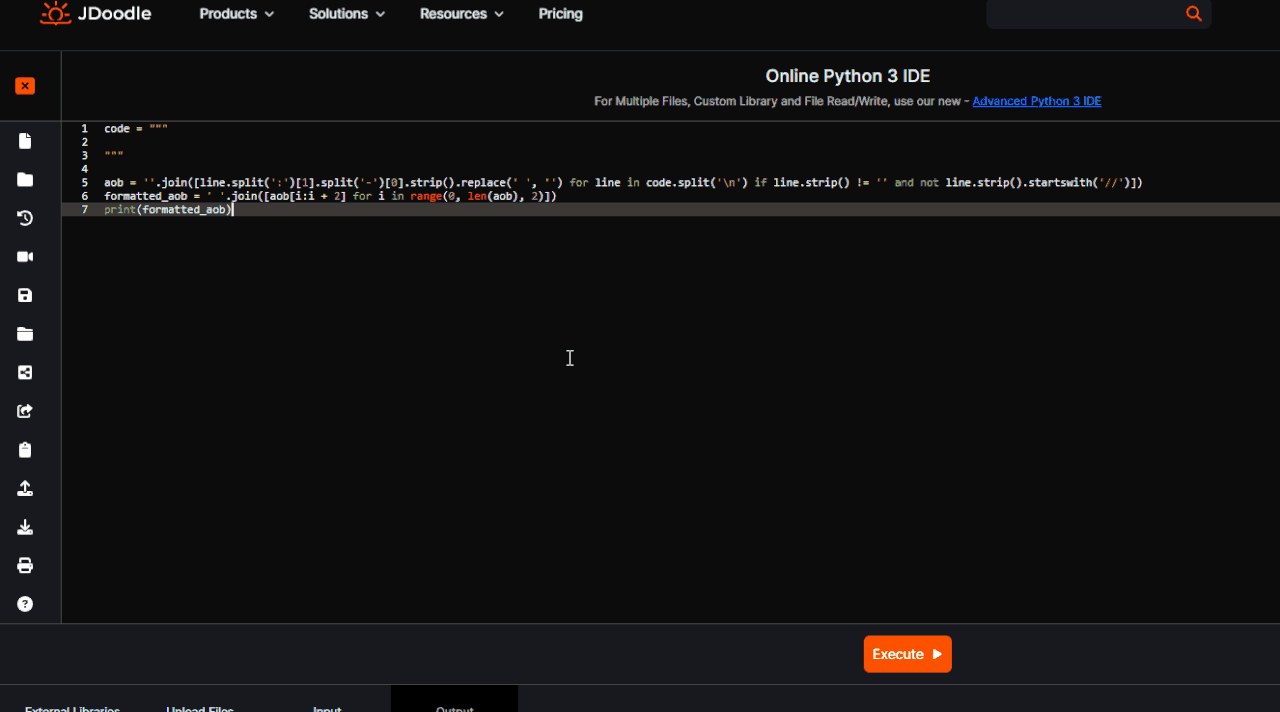
Spoiler
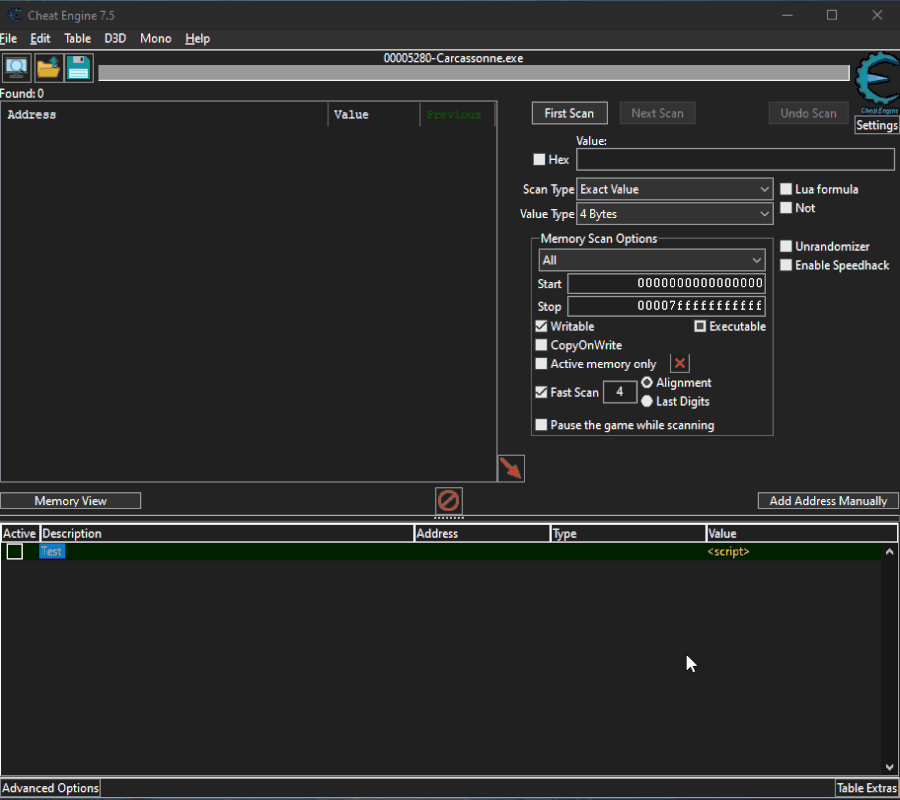
Spoiler
[Link] [Link] [Link]
I hope it is useful

Here is my Cheat Engine Tutorial:
[Link]