Hi Ash. Your codes work pretty well but I wonder if there is a way to assign the value of a stat <1 but >0 or >-1 but <0 ? There are stats like "more/less damage taken" but the lowest value i can assign it to is "1" which is 100% in game. I can't make it "99% damage taken" or lowerAsh06 wrote: ↑Thu Jun 08, 2023 12:33 pmCode: Select all
public static Il2CppSystem.Collections.Generic.List<UniqueItemMod> NameOfTheMod() { //Create new Array of UniqueItemMod Il2CppSystem.Collections.Generic.List<UniqueItemMod> mods = new Il2CppSystem.Collections.Generic.List<UniqueItemMod>(); //Add UniqueItemMod to Array mods.Add(new UniqueItemMod { type = BaseStats.ModType.INCREASED, value = 999, maxValue = 999, property = SP.AttackSpeed, tags = AT.None }); //Add More return mods; }
Last Epoch Save Editor
Re: Last Epoch Save Editor
-
- Noobzor
- Posts: 13
- Joined: Wed Apr 19, 2017 3:32 pm
- Reputation: 1
Re: Last Epoch Save Editor
Hey long,long13579 wrote: ↑Mon Jun 12, 2023 5:39 pmHi Ash. Your codes work pretty well but I wonder if there is a way to assign the value of a stat <1 but >0 or >-1 but <0 ? There are stats like "more/less damage taken" but the lowest value i can assign it to is "1" which is 100% in game. I can't make it "99% damage taken" or lowerAsh06 wrote: ↑Thu Jun 08, 2023 12:33 pmCode: Select all
public static Il2CppSystem.Collections.Generic.List<UniqueItemMod> NameOfTheMod() { //Create new Array of UniqueItemMod Il2CppSystem.Collections.Generic.List<UniqueItemMod> mods = new Il2CppSystem.Collections.Generic.List<UniqueItemMod>(); //Add UniqueItemMod to Array mods.Add(new UniqueItemMod { type = BaseStats.ModType.INCREASED, value = 999, maxValue = 999, property = SP.AttackSpeed, tags = AT.None }); //Add More return mods; }
you could try to add the value as a float, like this:
Code: Select all
mods.Add(new UniqueItemMod
{
type = BaseStats.ModType.INCREASED,
value = 0.9f,
maxValue = 0.99f,
property = SP.AttackSpeed,
tags = AT.None
});
Tell me if it worked for you!
Re: Last Epoch Save Editor
Thanks a lot. It work perfectly fine xDCyrexBabbel wrote: ↑Mon Jun 12, 2023 6:42 pm
Hey long,
you could try to add the value as a float, like this:
min value should be 90% and the max 99%.Code: Select all
mods.Add(new UniqueItemMod { type = BaseStats.ModType.INCREASED, value = 0.9f, maxValue = 0.99f, property = SP.AttackSpeed, tags = AT.None });
Tell me if it worked for you!
Re: Last Epoch Save Editor
Add = + flat value
Increase = % value with 1 =100%
Float min value is -1.
Try Increase with value like -0.5f for -50%
Last edited by Ash06 on Mon Jun 12, 2023 7:26 pm, edited 1 time in total.
Re: Last Epoch Save Editor
Thank you ! Your tools are fun xD
Re: Last Epoch Save Editor
It's nice to edit variables, but it's better if we use game functions.
Ex : Add Buffs to character (launch with F9)
Ex : game LevelUp function (launch with F10)
See github for this
Ex : Add Buffs to character (launch with F9)
Code: Select all
if (Input.GetKeyDown(KeyCode.F9)) //Exemple Buff Character
{
foreach (UnityEngine.Object obj in UniverseLib.RuntimeHelper.FindObjectsOfTypeAll(typeof(UnityEngine.Object)))
{
if ((obj.name == "MainPlayer(Clone)") && (obj.GetActualType() == typeof(StatBuffs)))
{
float duration = 255;
SP propertie = SP.Intelligence;
float added_value = 255;
float increase_value = 255;
Il2CppSystem.Collections.Generic.List<float> more_values = null;
AT tag = AT.Buff;
byte special_tag = 0;
obj.TryCast<StatBuffs>().addBuff(duration, propertie, added_value, increase_value, more_values, tag, special_tag);
}
}
}
Code: Select all
if (Input.GetKeyDown(KeyCode.F10))
{
foreach (UnityEngine.Object obj in UniverseLib.RuntimeHelper.FindObjectsOfTypeAll(typeof(UnityEngine.Object)))
{
if ((obj.name == "MainPlayer(Clone)") && (obj.GetActualType() == typeof(ExperienceTracker)))
{
obj.TryCast<ExperienceTracker>().LevelUp(true);
break;
}
}
}
Code: Select all
//ItemDrop
Mods.Character_Mods.increase_equipment_droprate = 1;
Mods.Character_Mods.increase_equipmentshards_droprate = 1;
Mods.Character_Mods.increase_uniques_droprate = 1;
//Speed Manager
Mods.Character_Mods.base_movement_speed = 10; //default 4,8
//Ability List
Mods.Character_Mods.channel_cost = 0;
Mods.Character_Mods.manaCost = 0;
Mods.Character_Mods.manaCostPerDistance = 0;
Mods.Character_Mods.minimumManaCost = 0;
Mods.Character_Mods.noManaRegenWhileChanneling = false;
Mods.Character_Mods.stopWhenOutOfMana = false;
//Tree Data
Mods.Character_Mods.CharacterClassID character_class = CharacterClassID.Sentinel;
Mods.Character_Mods.character_level = 100;
Mods.Character_Mods.chosen_mastery = 0;
Mods.Character_Mods.number_of_unlocked_slots = 5;
Mods.Character_Mods.passiveTree_pointsEarnt = 65535;
Mods.Character_Mods.skilltree_level = 255;
//GoldTracker
Mods.Character_Mods.gold_value = 99999999;
//CharacterStats
Mods.Character_Mods.attack_rate = 255;
Mods.Character_Mods.attributes_str = 99999999;
Mods.Character_Mods.attributes_int = 99999999;
Mods.Character_Mods.attributes_vita = 99999999;
Mods.Character_Mods.attributes_dext = 99999999;
Mods.Character_Mods.attributes_atte = 99999999;
//ExperienceTracker
Mods.Character_Mods.NextLevelExperience = 0;
-
- Noobzor
- Posts: 13
- Joined: Wed Apr 19, 2017 3:32 pm
- Reputation: 1
Re: Last Epoch Save Editor
Hey Ash,
would it be possible to add something like "elemental nova" ( or any ability/skill ) on hit/crit as an affix?
would it be possible to add something like "elemental nova" ( or any ability/skill ) on hit/crit as an affix?
Re: Last Epoch Save Editor
I think Paranoia Helmet is the better exemple because this item contain 3 Ability mods and have class_req
[Link]
Class_req can be found in base item (ItemList.EquipmentItem[type].subItems[id].classRequirement)
Get the unique_id with SaveEditor : 221
In the game, Paranoia can be found in UniqueList.uniques[221]
See the 3 mods with Ability
Don't require target
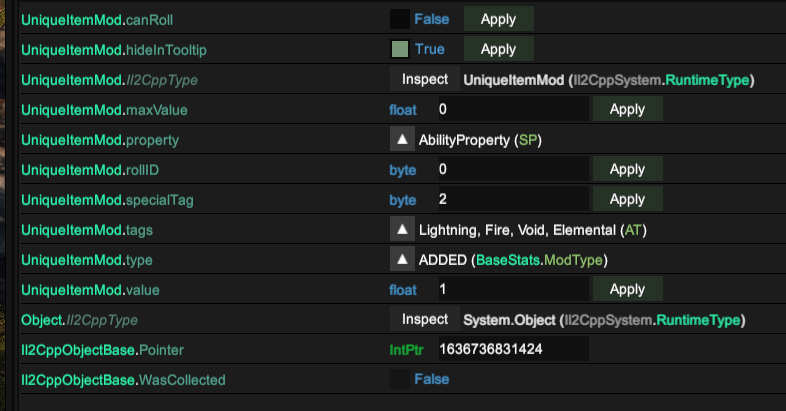
Cooldown Recovery
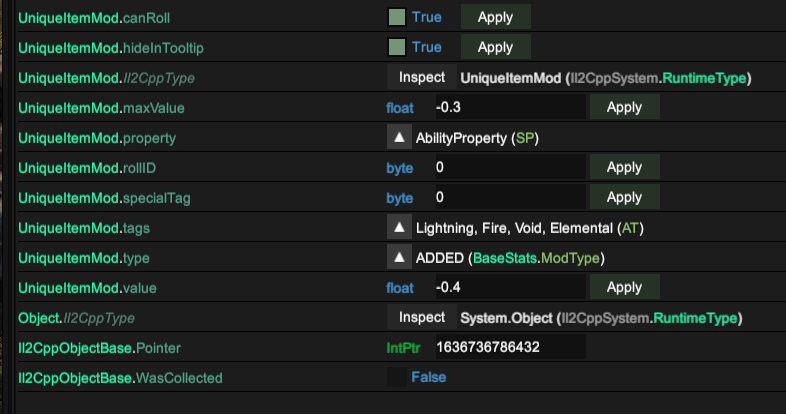
Level of skill
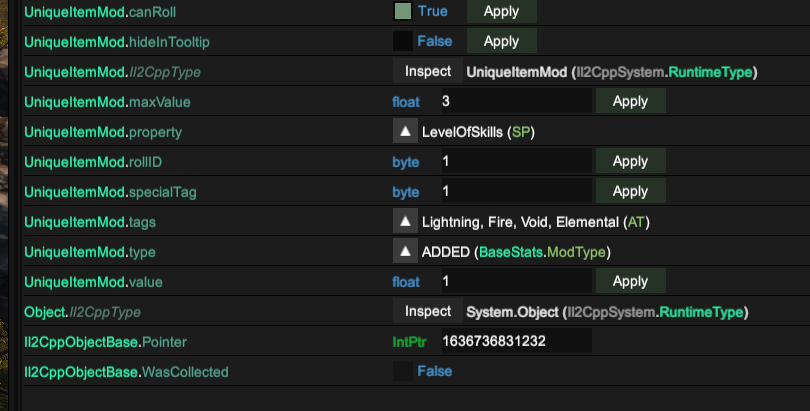
Special Tags can be found with this
Result for lunge

if you want custom ability on item, add some AbilityPropertyInfo
Skill_id, isn't in base_item but maybe in unique_item
[Link]
Class_req can be found in base item (ItemList.EquipmentItem[type].subItems[id].classRequirement)
Get the unique_id with SaveEditor : 221
In the game, Paranoia can be found in UniqueList.uniques[221]
See the 3 mods with Ability
Don't require target
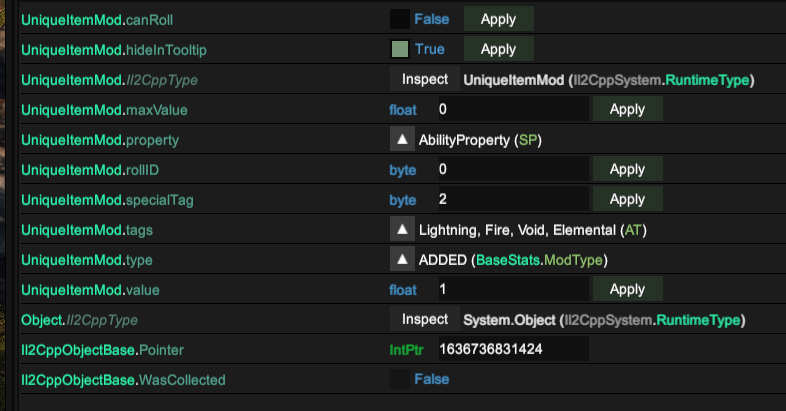
Cooldown Recovery
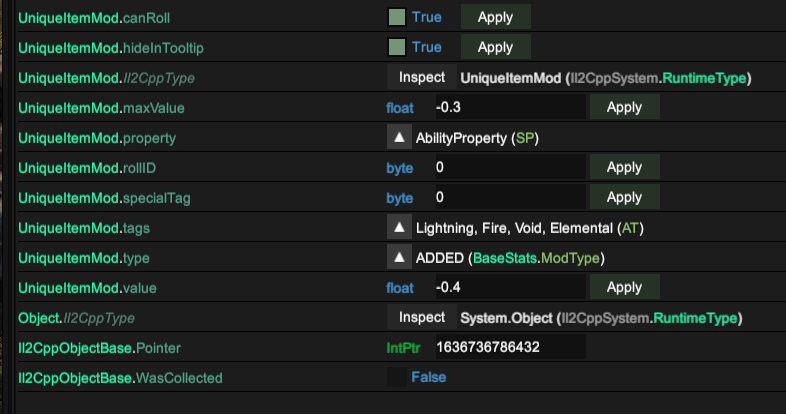
Level of skill
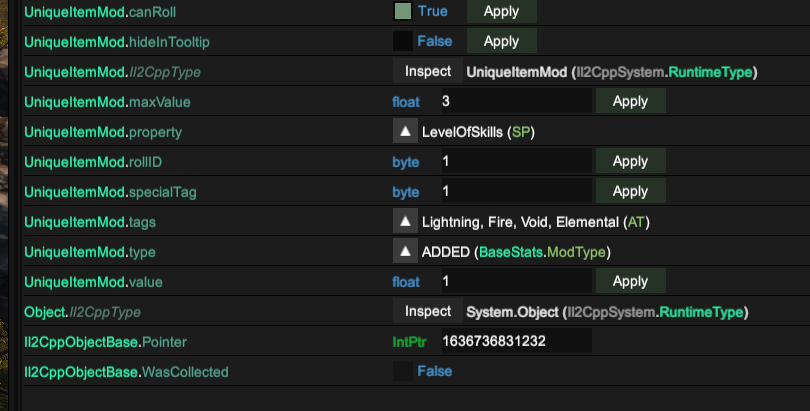
Special Tags can be found with this
Code: Select all
foreach (UnityEngine.Object obj in UniverseLib.RuntimeHelper.FindObjectsOfTypeAll(typeof(UnityEngine.Object)))
{
System.Type type = obj.GetActualType();
if ((type == typeof(AbilityPropertyList)) && (obj.name.Contains("AbilityPropertyList")))
{
AbilityPropertyList ability_property_list = obj.TryCast<AbilityPropertyList>();
int i = 0;
foreach (AbilityPropertyList.Entry entry in ability_property_list.list)
{
LoggerInstance.Msg(i + " : " + entry.abilityID);
int property_index = 0;
foreach (AbilityPropertyInfo ability_property_infos in entry.properties)
{
LoggerInstance.Msg(property_index + " : " + ability_property_infos.propertyName);
property_index++;
}
i++;
}
break;
}
}

if you want custom ability on item, add some AbilityPropertyInfo
Skill_id, isn't in base_item but maybe in unique_item
-
- Noobzor
- Posts: 13
- Joined: Wed Apr 19, 2017 3:32 pm
- Reputation: 1
Re: Last Epoch Save Editor
Hey Ash,
thanks again for your reply!
Got all the id´s but still having trouble to find where the skill_id is determined.
I looked into the unique item and also in the equipment list, but couldnt find anything useful ( at least with my basic knowledge ).
thanks again for your reply!
Got all the id´s but still having trouble to find where the skill_id is determined.
I looked into the unique item and also in the equipment list, but couldnt find anything useful ( at least with my basic knowledge ).
Re: Last Epoch Save Editor
Sorry not home this week, you have to use dnsspy and found function for loading items (append when you load your game), then hook with UnityExplorer.
Filter your results with an item with Ability on it, then see used variables, and were there are store.
Some object are desctuct after using, so it's better to patch functions than editing variables.
I search for this yesterday, found item mods, then I search for event and make stealing mods from rare and unique working.
So i create à Headhunter ^^
Filter your results with an item with Ability on it, then see used variables, and were there are store.
Some object are desctuct after using, so it's better to patch functions than editing variables.
I search for this yesterday, found item mods, then I search for event and make stealing mods from rare and unique working.
So i create à Headhunter ^^
-
- Noobzor
- Posts: 13
- Joined: Wed Apr 19, 2017 3:32 pm
- Reputation: 1
Re: Last Epoch Save Editor
All good, thank you for you help!Ash06 wrote: ↑Fri Jun 23, 2023 5:12 pmSorry not home this week, you have to use dnsspy and found function for loading items (append when you load your game), then hook with UnityExplorer.
Filter your results with an item with Ability on it, then see used variables, and were there are store.
Some object are desctuct after using, so it's better to patch functions than editing variables.
I search for this yesterday, found item mods, then I search for event and make stealing mods from rare and unique working.
So i create à Headhunter ^^
Ill try my best and will take a look

"I search for this yesterday, found item mods, then I search for event and make stealing mods from rare and unique working.
So i create à Headhunter ^^"
Haha, thats amazing

Re: Last Epoch Save Editor
I'm sorry but when I hit Dev mode button, there is "Dev mode: off" at the right-down corner of the screen, and no other button like your screen shot.
Re: Last Epoch Save Editor
You have to enable dev mode (Click on "Dev mode: off") after unlock it
-
- What is cheating?
- Posts: 1
- Joined: Wed Jun 14, 2023 1:06 am
- Reputation: 0
Re: Last Epoch Save Editor
Thanks a lot for maintaining this. It's been a lot of help.
I have the latest version and I encountered an error when trying to edit Gambit of an Erased Rogue with the save editor. I guess it has something to do with it being the highest Id number, 301? The other new uniques all were OK.
I have the latest version and I encountered an error when trying to edit Gambit of an Erased Rogue with the save editor. I guess it has something to do with it being the highest Id number, 301? The other new uniques all were OK.

Re: Last Epoch Save Editor
That's right, take this fix.
Who is online
Users browsing this forum: No registered users